Basic Python Programming for Beginners
-
This tutorial is intended to give beginners a feel for syntax and methods that Python uses.
All coding will be done on the Raspberry Pi..
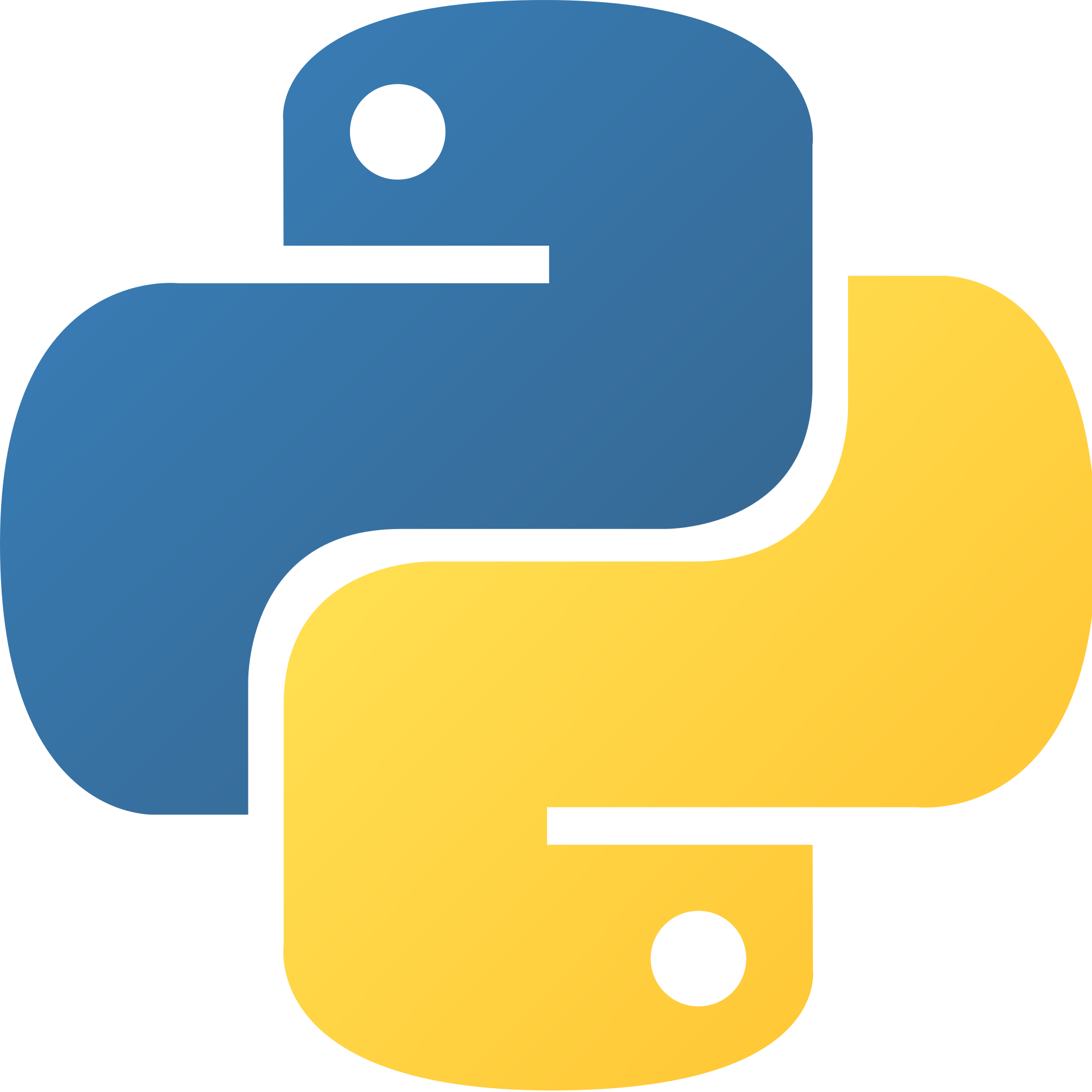
This tutorial assumes you have followed the lessons in the Getting Started section of the site.
You will need to have an internet connection set up as well.
You will need to have an internet connection set up as well.
Input/Output (I/O) and Variables
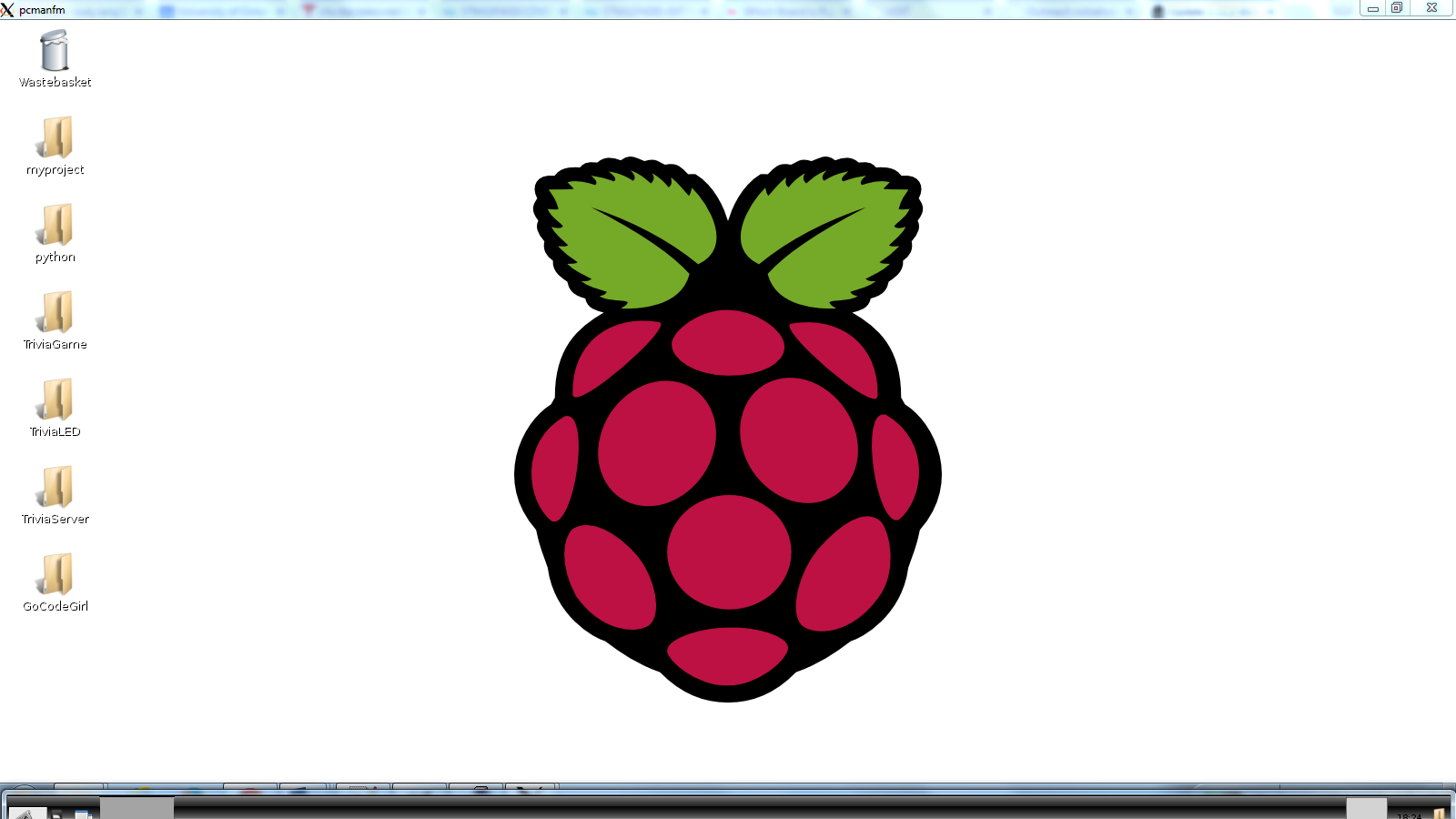
Boot up your Raspberry Pi and enter your login credentials (typically username: pi and password: raspberry).
Boot up the graphical desktop by typing
NOTE: Your desktop should look similar to the one on the left. It will look different depending on the version of Raspbian you are using, as well as the way you are accessing the Pi.
Boot up the graphical desktop by typing
startx
and hitting enter.
NOTE: Your desktop should look similar to the one on the left. It will look different depending on the version of Raspbian you are using, as well as the way you are accessing the Pi.
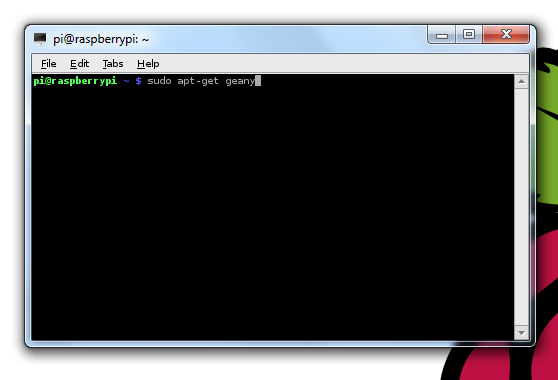
The first thing you will need to do is install Geany. Geany is the IDE (Integrated Development Environment) we will be using to write and run our code.
To install Geany, open up the LXTerminal which can be found by opening the Start menu and navigating to Accessories > LXTerminal.
Then, type:
To install Geany, open up the LXTerminal which can be found by opening the Start menu and navigating to Accessories > LXTerminal.
Then, type:
sudo apt-get install geany
and hit enter.
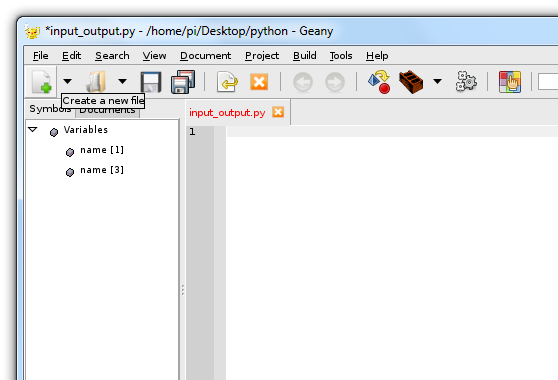
Once installed, you may access Geany navigating to Programming > Geany.
Once open, click the "new file" icon as shown on the right. Save the file by going to File > Save As.
Simply name the file "input_output.py" and click Save.
NOTE: The file name is arbitrary for this exercise; you may name it whatever you like.
NOTE: The file name is arbitrary for this exercise; you may name it whatever you like.
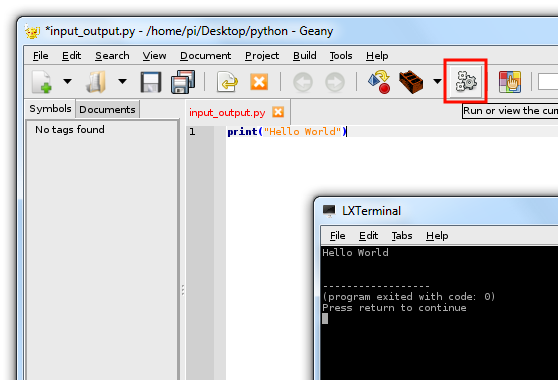
Now you can finally begin to program! In the window type
Print( ) is a built in function that displays whatever you have typed in the brackets. Once done, you may click the 3 gears to run the program in the console, as shown in the third figure to the right.
A console called the "LXTerminal" should appear with your phrase displayed at the top.
print("Hello World")
Print( ) is a built in function that displays whatever you have typed in the brackets. Once done, you may click the 3 gears to run the program in the console, as shown in the third figure to the right.
A console called the "LXTerminal" should appear with your phrase displayed at the top.
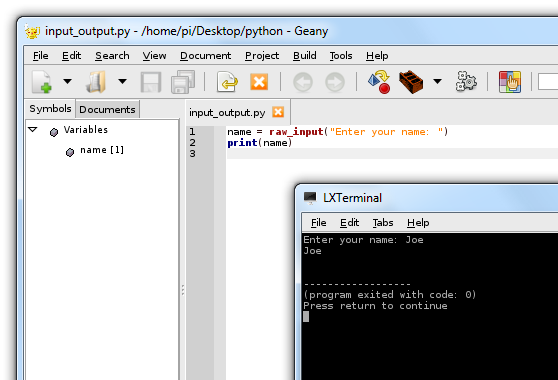
Next, we'll try to get user-entered input. To do this, we will use something called a variable. In programming, variables are used to store information in the form of a symbol.
That symbol is typically a letter or a word. Variables can be used multiple times throughout a program, which makes them useful for designing efficient applications.
Delete the print line from the window, and type
Now try running it by clicking the cogs on the top toolbar. Type in your name and hit Enter. Your name should be printed back to you!
name = raw_input("Enter your name: ")
followed by print(name)
on the following line.
It should look like code shown to the left.
Now try running it by clicking the cogs on the top toolbar. Type in your name and hit Enter. Your name should be printed back to you!
Conditions (if/else/elif)
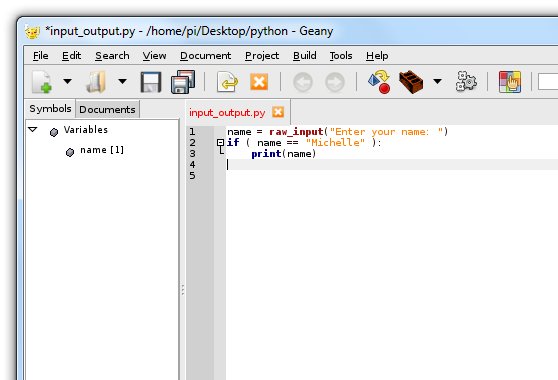
Next, we will cover conditional statements. These statements are used to create logic within a program. An IF statement is typically used to run a piece of code only if a specific condition is met. For instance, try the block of code on the left.
What this code is doing, is comparing the name you entered, to the name "Michelle". If the name you entered is in fact "Michelle", then that name will be printed. Otherwise, nothing will happen.
What this code is doing, is comparing the name you entered, to the name "Michelle". If the name you entered is in fact "Michelle", then that name will be printed. Otherwise, nothing will happen.
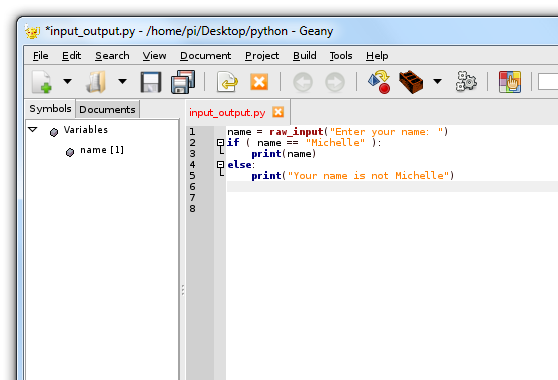
Now we'll add another statement, else. If the first condition is not met (your name isn't Michelle), then we'll print something else.
Add
The indentations after if and else are important; it implies that you only want to print if those specific conditions are met.
Another statement that you'll see is the else if statement. This statement is simply used to add additional conditions. A shorthand called
Add
else:
followed by print("Your name is not Michelle")
to end of your code. It should look like the figure to the left.
The indentations after if and else are important; it implies that you only want to print if those specific conditions are met.
Another statement that you'll see is the else if statement. This statement is simply used to add additional conditions. A shorthand called
elif():
(unique to python) is used to create this statement, and functions identically to the if statement.
Loops (for/while)
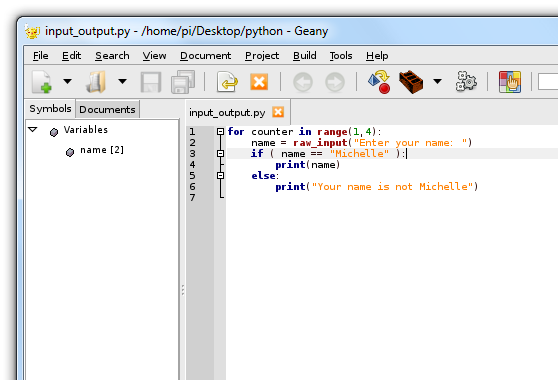
Loops are another important and widely used tool in programming. We will be focusing on two specific kinds of loops: for loops and while loops.
A for loop repeats a task for a set number of times, defined by a conditions. We are going to add a for loop to our current code so that it runs three times.
Add
In this case, the loop simply repeats the question four times.
A for loop repeats a task for a set number of times, defined by a conditions. We are going to add a for loop to our current code so that it runs three times.
Add
for counter in range(1, 4):
above all the previous code. Highlight all the code underneath and hit "tab". It is necessary to have this indent as it places our code inside the loop.
In this case, the loop simply repeats the question four times.
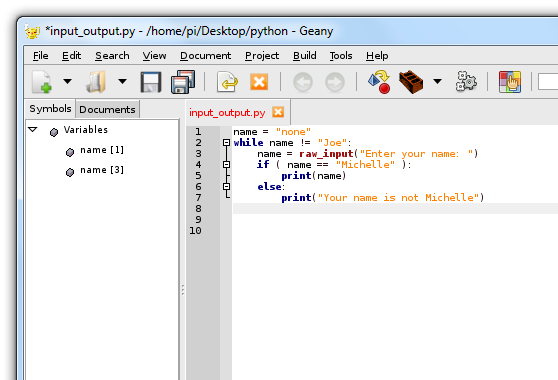
A while loop repeats until a specific condition is met. Let's modify our program so that it asks for our name until right one is entered.
Delete the for loop at the top and instead type
In this case, the loop will keep repeating the question until "Joe" is entered.
Delete the for loop at the top and instead type
name = "none"
, followed by while name != "_your name_":
, with the same code underneath.
In this case, the loop will keep repeating the question until "Joe" is entered.